
Are you an engineering student preparing for placements and have only 3 months at hand? This roadmap can help!!
Start by mastering a programming language and its collection framework or standard template library. Then, improve your data structures and algorithms skills by tackling medium and hard-level questions. Additionally, review essential computer science fundamentals. Finally, prepare for behavioral rounds and do your research on specific companies to impress potential employers. With this comprehensive approach, you'll be ready to excel in any engineering placement opportunity.
There’s a bonus at the end, don’t miss out on it :)
Jump ahead:
Phase 1: Programming Language Collections/ STL - 2 days Phase 2: DSA Practice - Easy and Medium interview questions - 38 days Phase 3: Hard interview questions and Web Development - 30 days Phase 4: CS Fundamentals - 10 days
Phase 1 - Programming Language Collections/ STL |
2 days
Basic understanding - Java/ Python/ C++
(Note. We are assuming you have a basic understanding of a language. If not then you would need at least 3 weeks to master any language and make sure you cover the below points in a chosen language, it could be Java, Python, or C++)
(Python: SkillYaan | Complete Python course)
To get a basic understanding of any programming language, you should cover the following topics:
Introduction:
Learn about the programming language's history, purpose, and unique features.
Understand the language's applications, community support, and popularity.
Environment Setup:
Learn how to install the necessary tools and software to write, compile, and run code in the language.
Understand how to use an Integrated Development Environment (IDE) or text editor for the language.
Syntax and Basic Concepts:
Learn the basic syntax rules and structure of the language.
Understand variables, data types, and operators.
Learn how to write and use functions or methods.
Understand how to handle errors and exceptions.
Control Structures:
Learn about conditional statements (if, else, and else-if) and how to use them to control program flow.
Understand looping structures (for, while, and do-while) and how to use them for iteration.
Data Structures and Collections:
Learn about the built-in data structures, such as arrays, lists, sets, dictionaries, and tuples.
Understand how to use these data structures to store and manipulate data.
Object-Oriented Programming (OOP): Link: SkillYaan | Object Oriented Programming
Learn about classes, objects, and inheritance.
Understand encapsulation, abstraction, and polymorphism.
Learn how to create and use instances of classes, and how to define and call class methods.
Input and Output (I/O):
Learn how to read from and write to the console, files, and other external sources.
Understand how to handle different types of input (e.g., command-line arguments, user input, or files).
Libraries and Modules:
Learn about the standard library and built-in modules for the language.
Understand how to import and use external libraries and modules to extend the language's functionality.
Learn writing clean and readable code:
Learn about coding conventions, style guides, and best practices for the language.
Understand the importance of writing clean, efficient, and maintainable code.
Basic Projects and Exercises:
Apply your knowledge by working on small-scale projects or coding exercises.
Collaborate with others, seek help from the community, and learn from online resources.
By covering these topics, you should gain a basic understanding of any programming language and be able to write simple programs. As you gain experience, you can explore more advanced topics and build increasingly complex projects.
Collection framework(Java)/ STL framework (C++) - 2 days
Both the Java Collection Framework and the Standard Template Library (STL) in C++ are libraries that provide data structures and algorithms to handle and manipulate data efficiently.
Here's an overview of each and some suggestions on how to learn them:
Java Collection Framework:
The Java Collection Framework is part of the Java Standard Library and provides interfaces, implementations, and algorithms to work with various data structures. The key components include:
Interfaces: Iterable, Collection, List, Set, Queue, Deque, Map
Implementations: ArrayList, LinkedList, HashSet, TreeSet, PriorityQueue, ArrayDeque, HashMap, TreeMap, LinkedHashMap, LinkedHashSet
Algorithms: Sorting, Searching
To learn the Java Collection Framework:
a. Understand the basic concepts and interfaces:
Start with the basics of Java Collections, such as Iterable, Collection, List, Set, Queue, Deque, and Map.
Learn the differences between each interface and their use cases.
b. Learn about the implementations:
Understand the differences between ArrayList, LinkedList, HashSet, TreeSet, PriorityQueue, ArrayDeque, HashMap, TreeMap, LinkedHashMap, and LinkedHashSet.
Study the performance characteristics of each implementation, such as time and space complexity, and when to use one over another.
c. Study the algorithms:
Learn about sorting, searching, and other algorithms provided by the Collections framework.
Understand how to use the Collections utility class to work with these algorithms.
d. Practice with examples and exercises:
Implement data structures and algorithms using the Java Collection Framework.
Work on exercises and projects to solidify your understanding and gain hands-on experience.
Sample Java Codes (by SkillYaan) accessible here: Here
e. Consult documentation and resources:
Refer to the official Java documentation for the Collections Framework.
Utilize online tutorials, videos, and courses for additional learning.
C++ Standard Template Library (STL):
The STL is a library in C++ that provides generic classes and functions for handling data structures and algorithms. The key components include:
Containers: Vector, List, Deque, Set, Multiset, Map, Multimap, Stack, Queue, Priority Queue
Algorithms: Sorting, Searching, Permutations, Transformations, etc.
Iterators: Input, Output, Forward, Bidirectional, Random Access
Functors: Function objects used in algorithms
To learn the STL in C++:
a. Understand the basic components:
Study the different containers, algorithms, iterators, and functors available in the STL.
Learn the differences between each container and their use cases.
b. Learn about the containers:
Understand the differences between Vector, List, Deque, Set, Map, Stack, Queue, and Priority Queue.
Study the performance characteristics of each container, such as time and space complexity, and when to use one over another.
c. Study the algorithms and iterators:
Learn about sorting, searching, and other algorithms provided by the STL.
Understand how to use iterators to work with algorithms and containers.
d. Practice with examples and exercises:
Implement data structures and algorithms using the C++ STL.
Work on exercises and projects to solidify your understanding and gain hands-on experience.
e. Consult documentation and resources:
Refer to the official C++ documentation for the STL.
Utilize online tutorials, videos, and courses for additional learning.
By following these steps and dedicating time to practice, you can learn both the Java Collection Framework and the C++ STL effectively.
Phase 2 - DSA Practice | 38 days
48 easy interview questions - 12 days
52 medium questions - 26 days
You can practise questions from any of the below lists -
Blind 75 Refer here
Skillyaan List Refer here
Neetcode 150 Refer here
Please ensure that for every topic you follow the below order:
Understand the concepts. You can find easy to understand explanations here
Solve Easy questions - Solidify the concept
Solve Medium level questions - Helps to improve problem solving skill
You're now done with DSA !!
Phase 3 - Web Development and Hard coding questions | 30 days
Web Dev project -
Develop a project using React JS . This will approximately take 4 weeks .You can use this playlist as starting point.
For 3 months of preparation, a Web Development project is realistic!! Yes, you read it right. Full Stack, Machine Learning would be a little heavy on you. It is worth noting that postings are more for React developers at a fresher level.
Web development is the process of building, creating, and maintaining websites and web applications. It is divided into two main categories:
Front-end (Client-side): Deals with the design, layout, and user interaction using HTML, CSS, and JavaScript.
Back-end (Server-side): Handles data storage, processing, and server-side logic using programming languages like Python, Ruby, PHP, or JavaScript (Node.js).
Looking to add some impressive projects to your resume that showcase your skills and potential? Here are some exciting project ideas that can help you stand out from the crowd!
Personal Portfolio: Want to create an online presence that showcases your abilities and interests? Build a personal portfolio website that highlights your skills, projects, and contact information. This is a great way to demonstrate your creativity, design skills, and ability to market yourself to potential employers.
Blog or Content Management System (CMS): Are you passionate about writing or sharing your knowledge with others? Develop a platform where you can publish articles, tutorials, or personal experiences. This will showcase your writing skills, as well as your ability to build and manage a website.
E-commerce Website: Do you have a knack for business and entrepreneurship? Build a fully-functioning online store to sell products or services. This will demonstrate your ability to create a user-friendly website, manage a database, and handle financial transactions.
Social Networking Site: Are you interested in creating communities and connecting people? Develop a social networking platform where users can interact, share, and communicate with one another. This will showcase your ability to create complex web applications and handle user data securely.
Online Booking System: Are you detail-oriented and organized? Develop a website for booking appointments, events, or services. This will demonstrate your ability to create a user-friendly interface, handle data securely, and manage complex scheduling algorithms.
Interactive Web Applications: Do you have a passion for front-end development and creating engaging user experiences? Build interactive tools, calculators, or games that demonstrate your creativity and technical skills.
RESTful API: Interested in backend development and serving data to other applications? Develop a server-side API to serve data for web applications or mobile apps. This will showcase your ability to design and implement a scalable and secure API.
Real-time Chat Application: Are you interested in real-time communication and messaging? Implement a real-time chat application using technologies like WebSockets or Firebase. This will showcase your ability to create real-time web applications and handle data securely.
Choose a project that aligns with your interests and skill set. Ensure it highlights your strengths and demonstrates your proficiency in web development technologies.
NOTE: DON’T LOSE HANG OF DSA - Leetcode Weekly Contests is a good way to keep practicing coding questions !
You can practise Hard level coding questions in this phase, try solving 1 in 2 days!!
Phase 4 - Computer Science Fundamentals | 10 days
Computer science fundamentals refer to the core concepts and principles that form the foundation of the field. Some topics that are important to cover in these fundamentals include:
Data structures: Organize and store data in a way that enables efficient access and modification. Examples include arrays, linked lists, stacks, queues, trees, and graphs.
Algorithms: Step-by-step procedures for solving problems or performing tasks, often applied to data structures. Topics include sorting (e.g., quicksort, merge sort), searching (e.g., binary search), and graph algorithms (e.g., Dijkstra's, breadth-first search).
Complexity analysis: Measures the efficiency of algorithms in terms of time and space requirements. This includes Big O notation, which helps compare and analyze the performance of different algorithms. (Link: SkillYaan | Time and Space Complexity Blog)
Programming paradigms: Approaches to organizing code and solving problems, such as imperative, functional, object-oriented, and declarative programming.
Computer architecture: The design and organization of computer systems, including topics such as the Von Neumann architecture, CPU components, memory hierarchy, and parallel processing.
Operating systems: Software that manages computer hardware and software resources, providing services for programs. Concepts include process management, memory management, file systems, and inter-process communication.
Networking: The study of how computers communicate and share information, covering topics such as the OSI model, network protocols (e.g., TCP/IP, HTTP), routing, and network security.
Databases: Systems for storing, retrieving, and managing data. Concepts include database design, normalization, SQL, and database management systems (e.g., relational, NoSQL).
Software engineering: The process of designing, developing, and maintaining software, involving best practices, methodologies (e.g., Agile, Waterfall), and tools (e.g., version control, testing, CI/CD).
Theory of computation: Explores the fundamental limits of computation and formal models, including topics such as automata theory, Turing machines, computability, and complexity classes (e.g., P, NP).
Artificial intelligence and machine learning: Techniques and algorithms for enabling computers to learn and make decisions, including topics like supervised learning, unsupervised learning, neural networks, and natural language processing.
These fundamentals provide a solid base for understanding and solving problems in computer science, and they serve as building blocks for more advanced concepts and applications.
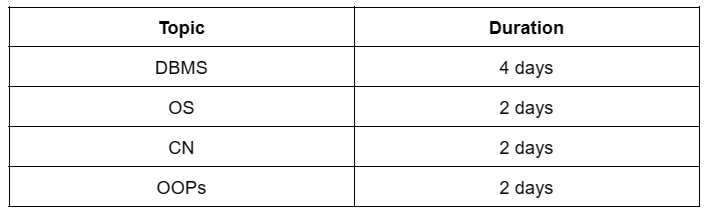
Phase 5 - Behavioral Round Prep and Resume creation | 2 days
Prepare for behavioral round - 1 day
Behavioral rounds in technical interviews assess interpersonal skills, teamwork, and cultural fit. They value collaboration, adaptability, and positivity in the work environment.
Interviewers ask about past experiences and hypothetical situations to gauge problem-solving approaches and communication abilities.
The goal is to understand behavior and potential reactions to future challenges.
Common topics covered in behavioral rounds include:
Teamwork and collaboration: Interviewers may ask about your experience working in teams, resolving conflicts, or collaborating with colleagues from diverse backgrounds.
Leadership: Questions may focus on instances where you took charge of a project, mentored teammates, or made critical decisions.
Communication: Interviewers may inquire about your ability to communicate complex ideas, present technical concepts to non-technical audiences, or handle difficult conversations.
Problem-solving and adaptability: You may be asked to describe situations where you faced challenges, developed innovative solutions, or adapted to changes in project requirements or team dynamics.
Time management and prioritization: Expect questions about meeting deadlines, handling multiple projects, and balancing work with other responsibilities.
Motivation and work ethic: Interviewers might ask about your passion for the field, your approach to continuous learning, or how you stay motivated during challenging times.
Cultural fit: Questions may address your alignment with the company's values, mission, and work environment, as well as your ability to contribute positively to the company culture.
To prepare for behavioral rounds, reflect on your experiences, and consider the STAR method (Situation, Task, Action, and Result) to structure your responses. Practice answering common behavioral questions, and be prepared to provide specific examples that demonstrate your skills and personal growth.
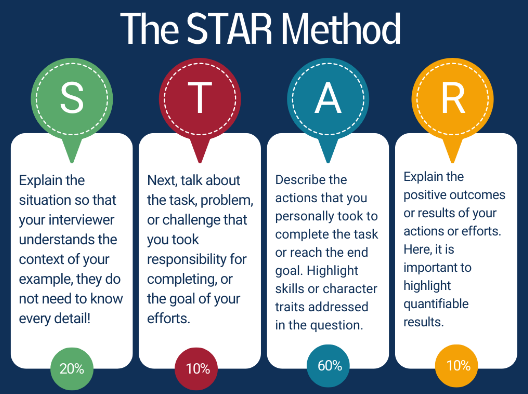
Source: MIT Career Services
Why do you want to work for us?
How do you handle a challenge?
What do you do if you disagree with someo/ne?
How do you prioritize tasks?
Have you worked on multiple projects?
Perfecting your resume - 1 day
A well-designed resume is crucial for making a strong first impression during the job application process, as it showcases your skills, experiences, and accomplishments in a clear and concise manner. To create an effective resume, it should be visually appealing, easy to read, and tailored to the specific job you are applying for. Start with a clean layout that highlights your most relevant experiences, using consistent formatting, appropriate font sizes, and sufficient white space to ensure readability. Emphasize your achievements by using action verbs, quantifying results when possible, and focusing on your impact in previous roles. Include a skills section to highlight relevant technical and soft skills, and customize your resume for each job application by emphasizing the experience and skills that best align with the position's requirements. A well-crafted resume is essential because it can significantly increase your chances of securing interviews, demonstrating your professionalism, and helping you stand out among other applicants.
Phase 6 - Company Specific Preparation | 15 days
Last 15 days prepare a list of 4 companies you want to get into and practice the questions asked by these companies in their interviews.
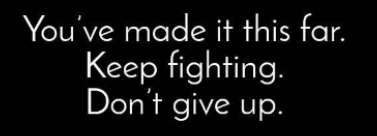
This step is crucial too, doing company-based preparation in the last few days enhances your chance to land a job.
Cherry on the cake:
SkillYaan | how-to-get-your-first-sde-job-in-product-based-companies-irrespective-of-your-background
Must also read: SkillYaan | How to Ace Coding Interviews
Placement Preparation Resources
Happy Learning!
Comments