Link to the video playlist: SkillYaan | Linked Lists
Link to the GitHub repository for Linked List codes: SkillYaan | Linked List
This blog has been divided into 3 sections, click on the buttons below to navigate:
Section 3 - What questions are to be asked in interviews? What are some good problems on Linked Lists one must prepare before interviews? Comparison of Linked Lists with other data structures and best practices to keep in mind when coding linked lists.
What is Linked Lists? What do they look like? How do we code them? What operations do we perform on them? Time and Space complexity chart.
What is a Linked List?
Linked lists are a dynamic and linear data structure used in computer science and engineering to store and manage collections of elements. They consist of nodes, each containing an element and a pointer to the next node. Linked lists can grow or shrink in size, making them more flexible than arrays. They also provide efficient insertion and deletion operations, making them useful for working with large data sets. However, they have slower access times and more memory overhead compared to arrays, which can be disadvantageous in some applications. Ultimately, the choice between using a linked list or an array depends on the specific needs of the application.

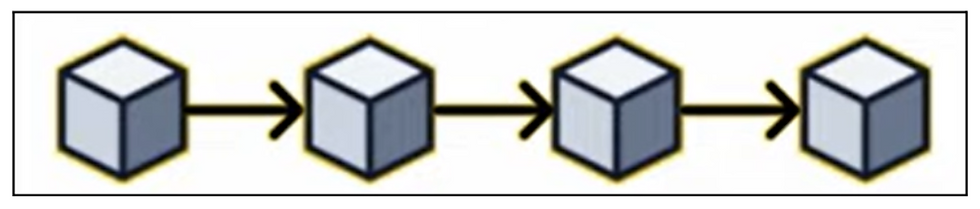
A linked list is a dynamic data structure that consists of a sequence of nodes, each containing an element and a pointer to the next node. Unlike arrays, which have a fixed size, linked lists can grow or shrink in size as elements are added or removed. Linked lists can be used to implement various abstract data types, such as lists, stacks, and queues.
The basic operations performed on a linked list include:
Insertion: Adding a new element to the list at a specific position.
Deletion: Removing an element from the list at a specific position.
Traversal: Visiting each element in the list in order.
Here is an example of a simple linked list:
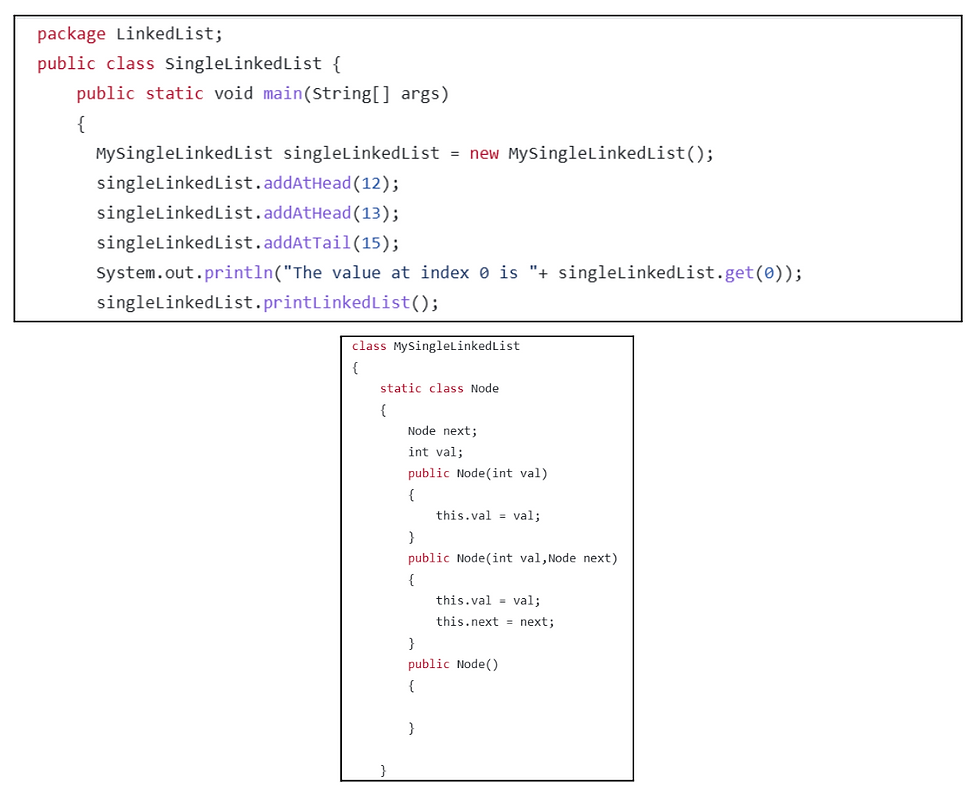
In this example, the linked list has three nodes with data values of 1, 2, and 3, respectively. The head variable points to the first node in the list.
Here are some advanced operations that can be performed on linked lists:
Merging two linked lists: Two linked lists can be merged into a single linked list by connecting the last node of the first list to the head node of the second list.
Reversing a linked list: The order of the elements in a linked list can be reversed by iterating through the list and updating the pointers of each node to point to the previous node instead of the next node.
Detecting a loop in a linked list: If a linked list contains a loop, it can be detected by using two pointers that traverse the list at different speeds. If the pointers meet at any point, then there is a loop in the list.
Finding the middle element of a linked list: The middle element of a linked list can be found by using two pointers that traverse the list at different speeds. When the faster pointer reaches the end of the list, the slower pointer will be pointing to the middle element.
Removing duplicates from a linked list: Duplicates can be removed from a linked list by iterating through the list and keeping track of the elements that have already been seen. If a duplicate element is encountered, it can be removed by updating the pointers of the previous node to point to the next node.
Partitioning a linked list: A linked list can be partitioned into two lists based on a given value by iterating through the list and adding nodes with values less than the given value to one list and nodes with values greater than or equal to the given value to another list.
Here is the time and space complexity chart for basic operations on a singly linked list:
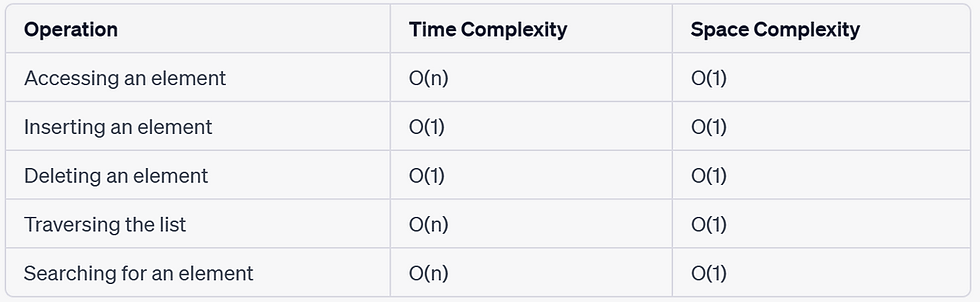
In a doubly linked list, accessing an element can be done in O(n/2) on average because you can traverse the list from either direction. Similarly, inserting and deleting elements can be done in O(1) because you can update the pointers in both the previous and next nodes.
In terms of space complexity, both singly and doubly linked lists require O(n) space to store the elements and node pointers.
It's important to note that these time and space complexities are for basic operations on a linked list. More complex operations, such as sorting or merging two linked lists, may have different time and space complexities depending on the specific algorithm used.
What are the applications of Linked Lists? What is the difference between them and arrays?
Linked lists have various applications in engineering. Here are a few examples:
File Systems: Linked lists are used to represent the directory structure in file systems. Each directory is represented by a node, and the pointer to the next node represents the subdirectories within that directory. This makes it easy for users to navigate through the files and directories in the system.
Music Players: Linked lists are used to implement playlists in music players. Each song is represented by a node, and the pointer to the next node represents the next song in the playlist. This allows users to easily add or remove songs from their playlists and play them in the desired order.
Operating Systems: Linked lists are used to manage memory in operating systems. Each block of memory is represented by a node, and the pointer to the next node represents the next block of memory. This allows the operating system to efficiently allocate and deallocate memory as needed by different processes.
Compiler Design: Linked lists are used to implement symbol tables in compilers. Each symbol is represented by a node, and the pointer to the next node represents the next symbol in the table. This allows the compiler to efficiently look up symbols and perform various operations on them.
Overall, linked lists are a versatile data structure that can be used in a wide range of engineering applications. Their dynamic nature and efficient memory management make them particularly useful in situations where the size of the data set is unknown or can change dynamically.
Let us look at the differences between Arrays and Linked Lists:-
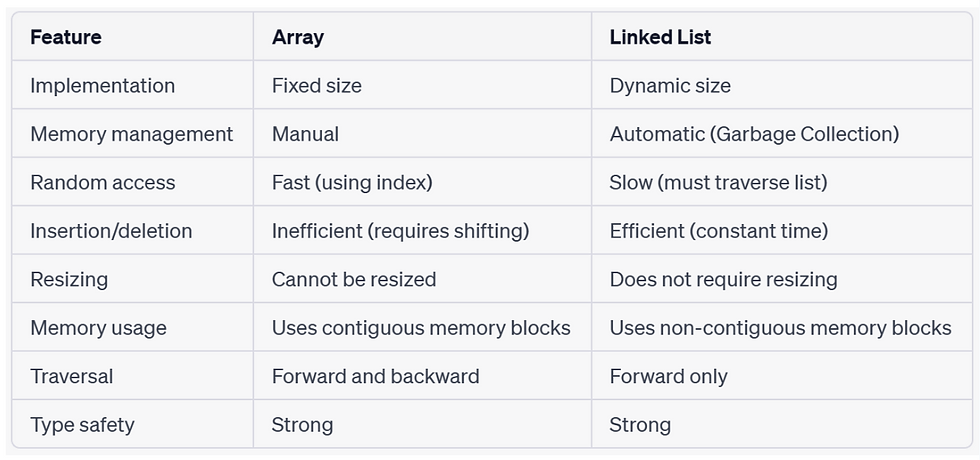
There are three main types of linked lists: singly linked lists, doubly linked lists, and circular linked lists. Here's a detailed explanation of each type:
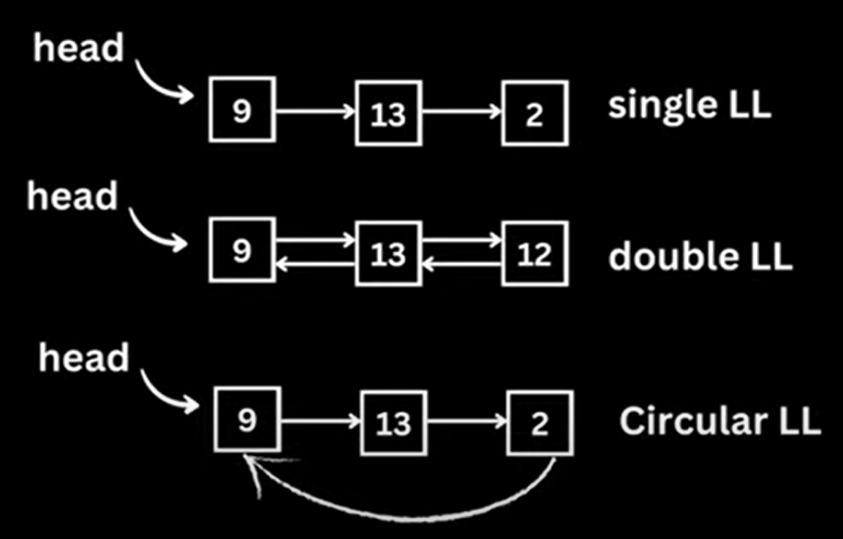
Singly Linked Lists:
A singly linked list is a type of linked list in which each node contains an element and a pointer to the next node. The last node in the list points to a null value, indicating the end of the list. Singly-linked lists are easy to implement and require less memory overhead compared to other types of linked lists. However, they do not support efficient traversal in reverse order and require extra effort to delete a node.
Doubly Linked Lists:
A doubly linked list is a type of linked list in which each node contains an element and two pointers: one pointing to the next node and the other pointing to the previous node. The first node in the list points to a null value for the previous node pointer, and the last node in the list points to a null value for the next node pointer. Doubly linked lists allow for efficient traversal in both forward and reverse directions, but they require more memory overhead than singly-linked lists due to the extra pointer in each node.
Circular Linked Lists:
A circular linked list is a type of linked list in which the last node in the list points to the first node, creating a circular loop. Each node contains an element and a pointer to the next node. Circular linked lists are useful for implementing applications where a continuous loop is needed, such as in video players or traffic light systems. However, they require extra care to ensure that the loop is correctly maintained and can be more complex to implement than other types of linked lists.
Overall, the choice of which type of linked list to use depends on the specific requirements of the application. Singly-linked lists are simple and efficient for many use cases, while doubly-linked lists provide additional flexibility for traversing the list in reverse order. Circular linked lists are useful for implementing loops and continuous cycles, but they can be more complex to implement and maintain.
What questions are to be asked in interviews? What are some good problems on Linked Lists one must prepare before interviews? Comparison of Linked Lists with other data structures and best practices to keep in mind when coding linked lists.
Here are a few questions related to linked lists that could be asked in engineering interviews for product-based companies:
What is a linked list and how is it different from an array?
What are the basic operations performed on a linked list?
What are the advantages and disadvantages of using a linked list instead of an array?
How would you implement a function to add a node to the beginning of a linked list?
How would you implement a function to remove the last node of a linked list?
How would you implement a function to find the middle node of a linked list?
What is a doubly linked list and how is it different from a singly linked list?
How would you implement a function to reverse a linked list?
How would you implement a function to detect if a linked list has a cycle?
How would you implement a function to merge two sorted linked lists into a single sorted list?
The question "What are the advantages and disadvantages of using a linked list instead of an array?" has not been answered yet.
Advantages of using a linked list over an array include:
Dynamic size: Linked lists can grow or shrink in size as elements are added or removed, whereas arrays have a fixed size that cannot be changed once they are created.
Efficient insertion and deletion: Linked lists provide efficient insertion and deletion operations, especially when working with large data sets. In contrast, arrays require shifting elements to make room for new ones, which can be time-consuming for large data sets.
Flexibility: Linked lists can be used to implement various data structures, such as stacks and queues, whereas arrays have limited flexibility in this regard.
Disadvantages of using a linked list over an array include:
Slower access times: Linked lists have slower access times compared to arrays because they do not provide direct access to elements. Instead, elements must be accessed sequentially by traversing the list.
More memory overhead: Linked lists require more memory overhead than arrays because each element must store a pointer to the next element. This can be a concern for large data sets where memory usage is critical.
No random access: Linked lists do not provide random access to elements, which can be a disadvantage in some applications. In contrast, arrays provide constant-time access to elements using their index.
Overall, the choice between using a linked list or an array depends on the specific requirements of the application. If dynamic size and efficient insertion and deletion are important, a linked list may be the better choice. If random access and memory usage are the primary concerns, an array may be more suitable.
Here's a quick comparison of linked lists with other data structures:
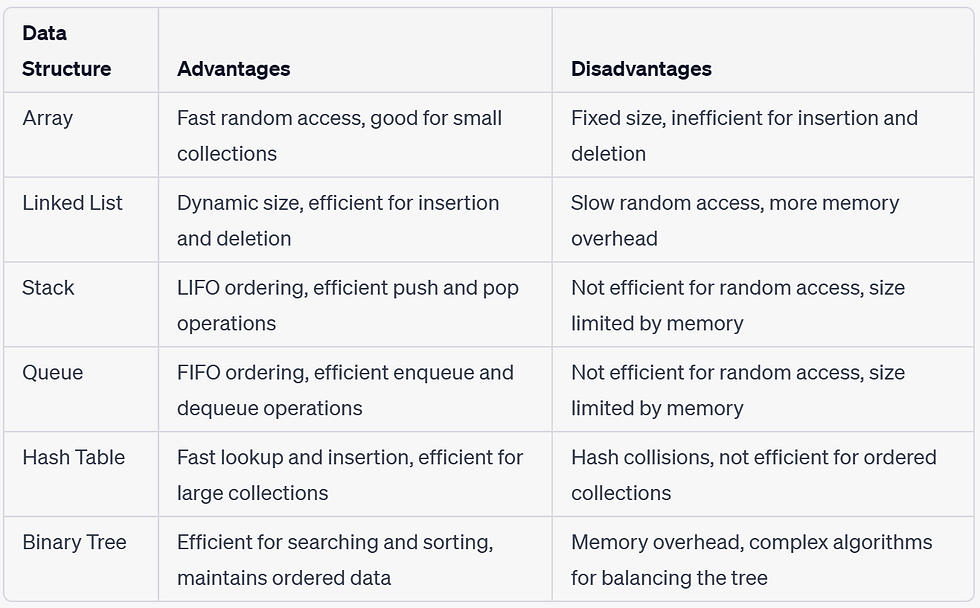
Here are some best practices to keep in mind when coding linked lists:
Use clear and descriptive variable names: Use variable names that are clear and descriptive to make your code more readable and maintainable.
Always check for null values: When traversing or manipulating a linked list, always check for null values to avoid NullPointerExceptions.
Implement defensive programming: Implement defensive programming techniques, such as null checks, input validation, and error handling, to prevent unexpected behavior and ensure the reliability of your code.
Consider memory usage: Linked lists can have higher memory usage than other data structures due to the extra memory needed for the node pointers. Consider the trade-off between memory usage and performance when deciding whether to use a linked list.
Avoid unnecessary node creations: Avoid creating unnecessary nodes when manipulating a linked list, as creating and deleting nodes can be costly in terms of time and memory.
Keep track of the head and tail pointers: Keep track of the head and tail pointers in a linked list to avoid traversal overhead when adding or removing nodes from either end of the list.
Test your code thoroughly: Test your linked list implementation thoroughly to ensure that it works as expected and handles edge cases properly.
Follow established coding standards: Follow established coding standards and style guides to make your code more readable and maintainable.
By following these best practices, you can ensure that your linked list implementation is efficient, reliable, and easy to understand and maintain.
Must-do Linked List coding questions for product-based companies
Read more from us: SkillYaan | Blogs
For more such insightful content on Software Engineering, Subscribe to our YouTube channel: SkillYaan | YouTube
Let us end on a humorous note,
Why did the linked list go to the gym? To work on its nodes!
Was this article helpful?
YES
NO
Comments